Wirecard Payment Page v1
Hosted Payment Page
Hosted Payment Page with Payment Method Selection
Integration is as easy as including a JavaScript library into the merchant’s checkout page:
<script src="https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"></script>
Specification of the payment data:
var requestedData = {
merchant_account_id: "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
request_id: "c68b9039-968d-1c6b-d9f6-27e9ab2bcb3e",
request_time_stamp: "20200116084718",
payment_method: "creditcard",
transaction_type: "authorization",
requested_amount: "2.56",
requested_amount_currency: "EUR",
locale: "en",
request_signature: "e44730486d180cca590bc2e8dea22bd175395636a37b0da0ef785"
}
A function call of that library provides the payment functionality:
WirecardPaymentPage.hostedPay(requestedData);
Or call a different name library with the same functionality:
ElasticPaymentPage.hostedPay(requestedData);
Sample: Redirecting to the Hosted Payment Page
<html>
<head>
<title>example 01</title>
<script src="https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"></script>
<script>
function pay() {
var requestData = {
"request_id": "4d87f443-423c-6b13-e609-cc8b59c32d6b",
"request_time_stamp": "20160817140742",
"merchant_account_id": "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"transaction_type": "auto-sale",
"requested_amount": "0",
"requested_amount_currency": "EUR",
"request_signature": "283a346275444f3f4fbbbd7e3bcf815b41c4edf1a2531208e201fab12a9b0d53",
"payment_method": "creditcard",
"first_name": "John",
"last_name": "Doe"
};
WirecardPaymentPage.hostedPay(requestData);
}
</script>
</head>
<body>
<h1>example 01</h1>
<form>
<input type="button" value="Pay" onClick="pay()">
</form>
</body>
</html>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>
Demo shop
</title>
<script src="https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"></script>
</head>
<body>
<form>
<input id="wirecard_pay_btn" type="button" onclick="pay()" value="Pay Now"> <script type="text/javascript">
function pay() {
var requestedData = {
merchant_account_id: "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
request_id: "c68b9039-968d-1c6b-d9f6-27e9ab2bcb3e",
request_time_stamp: "20150226084718",
payment_method: "creditcard",
transaction_type: "purchase",
requested_amount: "2.56",
requested_amount_currency: "EUR",
locale: "en",
request_signature: "kg44730486d159df0bc2e8dea22bd175395636a37b0da0ef785"
}
WirecardPaymentPage.hostedPay(requestedData);
}
</script>
</form>
</body>
</html>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>
Demo shop
</title>
<script src="https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"></script>
</head>
<body>
<form>
<input id="wirecard_pay_btn" type="button" onclick="pay()" value="Pay Now"> <script type="text/javascript">
function pay() {
var requestedData = {
merchant_account_id: "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
request_id: "c68b9039-968d-1c6b-d9f6-27e9ab2bcb3e",
request_time_stamp: "20150226084718",
payment_method: "paypal",
transaction_type: "debit",
requested_amount: "2.56",
requested_amount_currency: "EUR",
locale: "en",
request_signature: "d730486d159df0bc2e8dea22bd175395636a37b0da0ef785"
}
WirecardPaymentPage.hostedPay(requestedData);
}
</script>
</form>
</body>
</html>
Please note that only the fields payment_method and transaction_type differ.
|
Embedded Payment Page
From a technical point of view, merchants just include a JavaScript library:
<script src="https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"></script>
A function call of that library provides the payment functionality:
WirecardPaymentPage.embeddedPay(requestedData);
Or call a different name library with the same functionality:
ElasticPaymentPage.embeddedPay(requestedData);
Sample: Showing the Embedded Payment Page
<html>
<head>
<title>example 01</title>
<script src=" https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"/>
<script>
function pay() {
var requestData = {
"request_id" : "4d87f443-423c-6b13-e609-cc8b59c32d6b",
"request_time_stamp" : "20160817140742",
"merchant_account_id" : "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"transaction_type" : "auto-sale",
"requested_amount" : "0",
"requested_amount_currency" : "EUR",
"request_signature" : "283a346275444f3f4fbbbd7e3bcf815b41c4edf1a2531208e201fab12a9b0d53",
"payment_method" : "creditcard",
"first_name" : "John",
"last_name" : "Doe"
};
WirecardPaymentPage.embeddedPay(requestData);
}
</script>
</head>
<body>
<h1>example 01</h1>
<form>
<input type="button" value="Pay" onClick="pay()">
</form>
</body>
</html>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN">
<html>
<head>
<title>Demo shop</title>
<script src="https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"></script>
</head>
<body>
<input id="wirecard_pay_btn" type="button" onclick="pay()" value="Pay Now"/>
<script type="text/javascript">
function pay() {
var requestedData = {
merchant_account_id: "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
request_id: "c68b9039-968d-1c6b-d9f6-27e9ab2bcb3e",
request_time_stamp: "20150226084718",
payment_method: "creditcard",
transaction_type: "purchase",
requested_amount: "2.56",
requested_amount_currency: "EUR",
locale: "en",
request_signature: "e44730486d180cca590bc2e8dea22bd175395636a37b0da0ef785"
}
WirecardPaymentPage.embeddedPay(requestedData);
}
</script>
</body>
</html>
Seamless Integration
Integration is done via a JavaScript library which merchants include in their checkout page:
<script src="https://api-test.wirecard.com/engine/hpp/paymentPageLoader.js" type="text/javascript"></script>
There are three functions provided by the library:
-
Render the form:
seamlessRenderForm()
-
Submit the form:
seamlessSubmitForm()
-
Submit a payment request:
seamlessPay()
You can optionally use ElasticPaymentPage instead of WirecardPaymentPage , e.g. call ElasticPaymentPage.seamlessRenderForm() .
|
WirecardPaymentPage.seamlessRenderForm({
requestData : requestData, (1)
wrappingDivId : "seamless-target", (2)
onSuccess : processSucceededResult (3)
onError : processErrorResult (4)
});
Renders the form. Parameters are
1 |
requestData : request data object, same as for Hosted Payment Page and similar to REST API integration |
2 |
wrappingDivId : ID of the HTML element where the form will be rendered |
3 |
onSuccess : callback on successful render |
4 |
onError : callback if an error occurred |
WirecardPaymentPage.seamlessSubmitForm({
requestData : requestData, (1)
onSuccess : processSucceededResult, (2)
onError : processErrorResult (3)
});
Submits the form. Parameters are
1 |
requestData : additional request data (optional) |
2 |
onSuccess : callback on successful form submission |
3 |
onError : callback when an error occurred during form submission |
WirecardPaymentPage.seamlessPay({
requestData : requestData, (1)
onSuccess : processSucceededResult, (2)
onError : processErrorResult (3)
});
Submits the payment request. Parameters are
1 |
requestData : request data object, same as for Hosted Payment Page and similar to REST API integration |
2 |
onSuccess : callback on successful payment request submission |
3 |
onError : callback if an error occurred during payment request submission |
The only parameter of the functions in case of both success and error is response .
|
Card Form Integration
This use-case covers the payment form displayed directly on the merchant’s checkout page for a seamless user experience (no browser redirects).
Render Form
Merchant renders the form into element on their checkout page. The
transaction details are specified in requestData
. The element is
identified by wrappingDivId
parameter.
var requestData = {
"request_id" : "217c3832-8575-c1d5-0e3a-2fa08003b0fd",
"request_time_stamp" : "20190403095835",
"merchant_account_id" : "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"transaction_type" : "purchase",
"requested_amount" : "2.00",
"requested_amount_currency" : "EUR",
"ip_address" : "127.0.0.1",
"request_signature" : "43fd8c261a8fdad693024c7cc009239ad344f1e5716de0c3163237b5392d5700",
"payment_method" : "creditcard"
};
WirecardPaymentPage.seamlessRenderForm({
requestData : requestData,
wrappingDivId : "seamless-target",
onSuccess : processSucceededResult,
onError : processErrorResult
});
Use a unique Request ID for each request. |
{
"ip_address": "127.0.0.1",
"merchant_account_id": "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"request_id": "217c3832-8575-c1d5-0e3a-2fa08003b0fd",
"requested_amount": "2.00",
"requested_amount_currency": "EUR",
"status_code_1": "201.0000",
"status_description_1": "The resource was successfully created.",
"status_severity_1": "information",
"transaction_id": "5ff1cf52-8471-11e5-95ea-005056b13376",
"transaction_state": "success",
"transaction_type": "check-signature"
}
Submit Form
Merchant assigns the call function to a pay button on the checkout page.
requestData
are optional when submitting form. They will be merged with
data from the first step. Form input is validated before the submit.
WirecardPaymentPage.seamlessSubmitForm({
onSuccess : processSucceededResult,
onError : processErrorResult
});
{
"api_id": "elastic-api",
"authorization_code": "153620",
"completion_time_stamp": "20190406103540",
"first_name": "John",
"ip_address": "127.0.0.1",
"last_name": "Doe",
"masked_account_number": "444433******1111",
"merchant_account_id": "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"payment_method": "creditcard",
"provider_transaction_id_1": "8ee6e543-c96f-459a-a379-50d0e9152fd3",
"request_id": "217c3832-8575-c1d5-0e3a-2fa08003b0fd",
"requested_amount": "2.00",
"requested_amount_currency": "EUR",
"self": "https://api-test.wirecard.com:9000/engine/rest/merchants/61e8c484-dbb3-4b69-ad8f-706f13ca141b/payments/1fe8a33a-8472-11e5-95ea-005056b13376",
"status_code_1": "201.0000",
"status_description_1": "3d-acquirer:The resource was successfully created.",
"status_severity_1": "information",
"token_id": "4186409015611111",
"transaction_id": "1fe8a33a-8472-11e5-95ea-005056b13376",
"transaction_state": "success",
"transaction_type": "purchase"
}
Decoupling Card Data Collection from Payment
When the merchant decides to display a recap order page between the payment data collection page and actually submitting the payment, the merchant may use the payment form to perform a:
-
Zero authorization transaction that may later be referenced by a regular authorization or purchase. The advantage for credit card payments is that a zero authorization transaction is confirmed by an issuer and the CVC is verified.
-
Tokenization-only transaction. A token (non-sensitive data) is returned to the merchant that can be used for a real authorization or purchase later.
In either case, sensitive payment data will not touch any of the merchant’s systems.
The difference to the previous scenario is that an authorization-only
transaction type with a zero amount is used instead of a purchase
transaction type (tokenization
transaction type may be used for a
similar effect), and the real fund booking only comes in the third
(additional) step. The payment form is displayed directly on the merchant’s
checkout page.
Render Form
The merchant renders the form into an element on their checkout page. The
transaction details are specified in requestData
. The element is
identified by wrappingDivId
parameter.
var requestData = {
"request_id" : "217c3832-8575-c1d5-0e3a-2fa08003b0fd",
"request_time_stamp" : "20190403095835",
"merchant_account_id" : "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"transaction_type" : "authorization-only",
"requested_amount" : "0",
"requested_amount_currency" : "EUR",
"ip_address" : "127.0.0.1",
"request_signature" : "43fd8c261a8fdad693024c7cc009239ad344f1e5716de0c3163237b5392d5700",
"payment_method" : "creditcard"
};
WirecardPaymentPage.seamlessRenderForm({
requestData : requestData,
wrappingDivId : "seamless-target",
onSuccess : processSucceededResult,
onError : processErrorResult
});
Use a unique Request ID for each request. |
{
"ip_address": "127.0.0.1",
"merchant_account_id": "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"request_id": "217c3832-8575-c1d5-0e3a-2fa08003b0fd",
"requested_amount": "0",
"requested_amount_currency": "EUR",
"status_code_1": "201.0000",
"status_description_1": "The resource was successfully created.",
"status_severity_1": "information",
"transaction_id": "5ff1cf52-8471-11e5-95ea-005056b13376",
"transaction_state": "success",
"transaction_type": "check-signature"
}
Submit Form
The merchant will assign call of the function to a pay button on their
checkout page. requestData
are optional when submitting the form. They will
be merged with data from the first step. Form input is validated before
the submit.
WirecardPaymentPage.seamlessSubmitForm({
onSuccess : processSucceededResult,
onError : processErrorResult
});
{
"api_id":"elastic-api",
"authorization_code":"153620",
"completion_time_stamp":"20190406103540",
"first_name":"John",
"ip_address":"127.0.0.1",
"last_name":"Doe",
"masked_account_number":"444433******1111",
"merchant_account_id":"61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"payment_method":"creditcard",
"provider_transaction_id_1":"8ee6e543-c96f-459a-a379-50d0e9152fd3",
"request_id":"217c3832-8575-c1d5-0e3a-2fa08003b0fd",
"requested_amount":"0",
"requested_amount_currency":"EUR",
"self":"https://api-test.wirecard.com:9000/engine/rest/merchants/61e8c484-dbb3-4b69-ad8f-706f13ca141b/payments/1fe8a33a-8472-11e5-95ea-005056b13376",
"status_code_1":"201.0000",
"status_description_1":"3d-acquirer:The resource was successfully created.",
"status_severity_1":"information",
"token_id":"4186409015611111",
"transaction_id":"1fe8a33a-8472-11e5-95ea-005056b13376",
"transaction_state":"success",
"transaction_type":"authorization-only"
}
Actual Payment
The merchant can now reference an existing authorization-only transaction to do a payment without further consumer involvement. The merchant may also do this using the function from Wirecard’s JavaScript library from the browser.
var requestData = {
"request_id" : "679047dc-8a4d-657b-91dc-df6d80cd6a10",
"request_time_stamp" : "20190403101310",
"merchant_account_id" : "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"transaction_type" : "purchase",
"requested_amount" : "12",
"requested_amount_currency" : "EUR",
"ip_address" : "127.0.0.1",
"request_signature" : "ea468004287bf191a43b4c5ac33e38d5f035050ad784cad293646c1533e7fc48",
"payment_method" : "creditcard",
"parent_transaction_id" : "26c14051-8213-11e5-a96e-0050b667eb91"
};
WirecardPaymentPage.seamlessPay({
requestData : requestData,
onSuccess : processSucceededResult,
onError : processErrorResult
});
Use a unique Request ID for each request. |
{
"api_id": "elastic-api",
"authorization_code": "153620",
"completion_time_stamp": "20190406103540",
"first_name": "John",
"ip_address": "127.0.0.1",
"last_name": "Doe",
"masked_account_number": "444433******1111",
"merchant_account_id": "61e8c484-dbb3-4b69-ad8f-706f13ca141b",
"payment_method": "creditcard",
"provider_transaction_id_1": "8ee6e543-c96f-459a-a379-50d0e9152fd3",
"request_id": "217c3832-8575-c1d5-0e3a-2fa08003b0fd",
"requested_amount": "12",
"requested_amount_currency": "EUR",
"self": "https://api-test.wirecard.com:9000/engine/rest/merchants/61e8c484-dbb3-4b69-ad8f-706f13ca141b/payments/1fe8a33a-8472-11e5-95ea-005056b13376",
"status_code_1": "201.0000",
"status_description_1": "3d-acquirer:The resource was successfully created.",
"status_severity_1": "information",
"token_id": "4186409015611111",
"transaction_id": "1fe8a33a-8472-11e5-95ea-005056b13376",
"transaction_state": "success",
"transaction_type": "purchase"
}
Validation, Language and Custom Templates
Form Validation
Merchant can request form validity by using function.
The only parameter is the name of the callback function.
WirecardPaymentPage.seamlessFormIsValid({
onValidationResult : processValidationResult
});
Merchant can validate the form input anytime calling function.
The only parameter is the name of the callback function. Otherwise, the form is validated always on form submit.
WirecardPaymentPage.seamlessValidateForm({
onValidationResult : processValidationResult
});
Change Language
Merchant can change form’s locale using function.
The only parameter is the language ISO code.
WirecardPaymentPage.seamlessChangeLocale("en");
Custom Templates
Merchants are able to specify their own custom templates to be used
instead of Wirecard’s default payment form. The template consists of
HTML content, CSS and JavaScript parts. The following template is an
example of a merchant’s custom template that uses bootstrap and
form validation libraries. First, the HTML part is inserted into the <body>
tag of the page, so it is a simple HTML form:
<h3 data-i18n="cc_form_title">Billing information</h3>
<form id="seamless-form" data-wd-validate-form="true" th:object="${payment}">
<div class="row">
<div class="form-group col-xs-12">
<div class="card-types btn-group" data-toggle="buttons">
<label class="btn btn-default active"> <input type="radio"
class="ee-request-nvp" name="card_type" value="visa"
checked="checked" data-fv-wdcardtype="true"
data-fv-wdcardtype-message="select_valid_card_type"
data-fv-wdcardtype-creditcardfield="account_number" /> <img
src="https://upload.wikimedia.org/wikipedia/commons/thumb/5/5e/Visa_Inc._logo.svg/1280px-Visa_Inc._logo.svg.png"
alt="Visa" />
</label> <label class="btn btn-default"> <input type="radio"
class="ee-request-nvp" name="card_type" value="mastercard" /> <img
src="https://lh5.googleusercontent.com/-_o1E3Ie8C8Y/U1YW_49rhBI/AAAAAAAAAbI/vGfq1_kakSU/w800-h800/mastercard-logo-in-jokerman-font.png"
alt="Mastercard" />
</label> <label class="btn btn-default"> <input type="radio"
class="ee-request-nvp" name="card_type" value="diners" /> <img
src="http://www.golf.co.nz/uploads/diners-logo-transparent.png"
alt="Diners" />
</label>
</div>
</div>
</div>
<div class="row">
<div class="col-sm-8">
<div class="form-group">
<input type="text" class="form-control ee-request-nvp"
id="account_number" name="account_number" placeholder="Card number"
data-i18n="card_number" data-fv-wdcreditcard="true"
data-fv-wdcreditcard-message="enter_creditcard_number"
data-fv-wdcreditcard-cardtypefield="card_type"
data-fv-wdcreditcard-cvvfield="card_security_code"
data-fv-wdcreditcard-allowedcardtypes="visa,mastercard,diners"
data-fv-onsuccess="FormValidation.WDHelper.onCardNumberSuccess"
data-fv-onerror="FormValidation.WDHelper.onCardNumberError" />
</div>
</div>
<div class="col-sm-4">
<div class="form-group">
<input type="text" class="form-control ee-request-nvp"
id="card_security_code" name="card_security_code" placeholder="CVV"
data-fv-wdcvv="true" data-fv-wdcvv-message="enter_cvv"
data-fv-wdcvv-creditcardfield="account_number"
data-i18n="card_security_code" />
</div>
</div>
</div>
<div id="expiry-date-div" class="row">
<div class="col-sm-8"></div>
<div class="col-sm-2">
<div class="form-group">
<input type="number" class="form-control ee-request-nvp"
id="expiration_month" name="expiration_month" placeholder="Month"
data-i18n="expiration_month" data-fv-notempty="true"
data-fv-notempty-message="enter_value" data-fv-wdexpirymonth="true"
data-fv-wdexpirymonth-message="invalid_expiry_date"
data-fv-wdexpirymonth-yearfield="expiration_year" />
</div>
</div>
<div class="col-sm-2">
<div class="form-group">
<input type="number" class="form-control ee-request-nvp"
id="expiration_year" data-fv-notempty="true"
data-fv-notempty-message="enter_value" data-fv-wdexpiryyear="true"
data-fv-wdexpiryyear-message="invalid_expiry_date"
data-fv-wdexpiryyear-yearscount="20"
data-fv-wdexpiryyear-monthfield="expiration_month"
name="expiration_year" placeholder="Year"
data-i18n="expiration_year" />
</div>
</div>
</div>
<div class="row">
<div class="col-sm-6">
<div class="form-group">
<input type="text" class="form-control ee-request-nvp"
id="first_name" name="first_name"
placeholder="Cardholder first name" data-i18n="first_name"
th:value="*{accountHolder} ? *{accountHolder.firstName}" />
</div>
</div>
<div class="col-sm-6">
<div class="form-group">
<input type="text" class="form-control ee-request-nvp"
id="last_name" name="last_name" placeholder="Cardholder last name"
data-fv-notempty="true" data-fv-notempty-message="enter_value"
data-i18n="last_name"
th:value="*{accountHolder} ? *{accountHolder.lastName}" />
</div>
</div>
</div>
</form>
The HTML part may be completed with a CSS stylesheet:
body {
font-family: Verdana;
font-size: 12px;
padding: 20px;
}
input {
width: 100%;
}
.btn {
outline: none !important;
}
.card-types .btn {
height: 40px
}
.card-types .btn img {
width: 40px;
}
.card-types .form-control-feedback {
right: -40px !important;
top: 1px !important;
}
Each merchant may provide multiple templates and specify which one to use when rendering the Seamless form.
Please contact merchant support in order to set up custom templates. |
Seamless Configuration UI
The Seamless Configuration UI endpoint has been created for the purpose of changing a Seamless template online and previewing it just in time.
After browser authentication, the Seamless Configuration UI is
accessible on
https://api-test.wirecard.com/engine/rest/config/seamless/ui
The following view should be displayed, once access is granted:
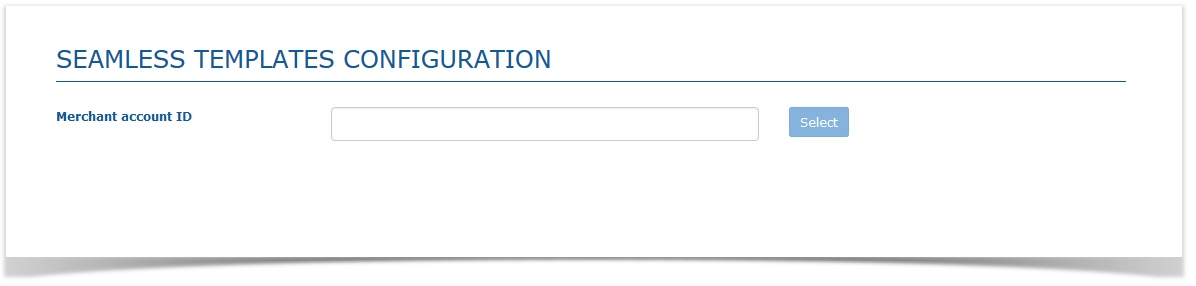
When an existing merchant is selected, all the merchant’s related templates should be loaded automatically from the server via REST API. The list of the merchant’s configured templates is displayed on the left-side menu. Template-specific details are displayed on the right in tabs.
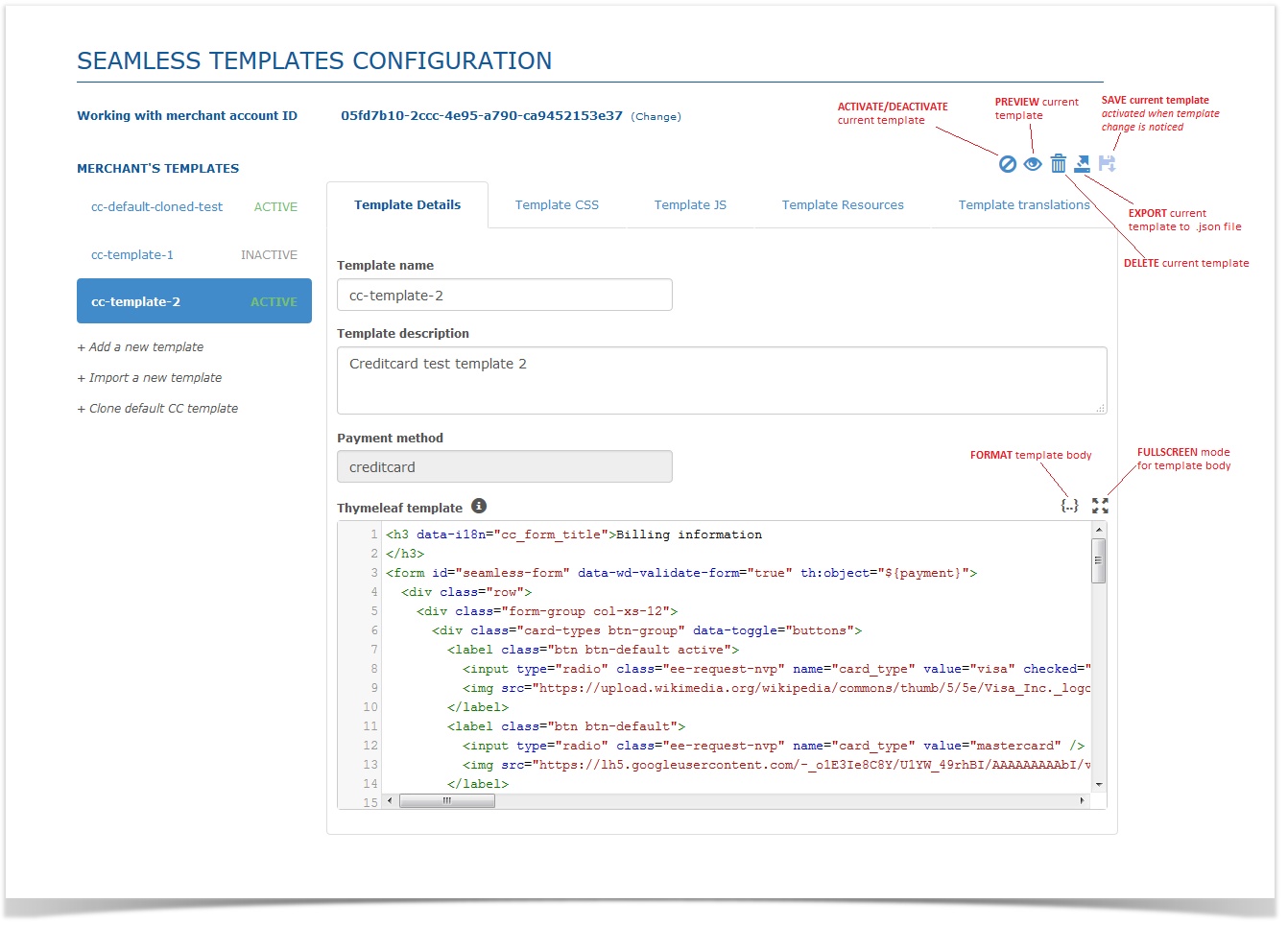
Production Deployment Process
Currently, the Seamless UI editor is available only on the TEST environment. Therefore, once a template has been created by the merchant and is ready for production, the merchant has to export the theme and send it to merchant support to upload it to the PRODUCTION environment.
Resources Management
The screenshot below shows the Template Resources (JS and CSS) configuration. Selecting resources is optional. A resource should be added just in case it is required by a particular template.
To select a resource, double-click on it in the Available Resources list on top (or select it and use arrows between list boxes). To deselect a resource, double-click on it in the bottom list of Selected Resources. To change the order, use the arrows next to the Selected Resources list.
The order is important when loading libraries with dependencies.
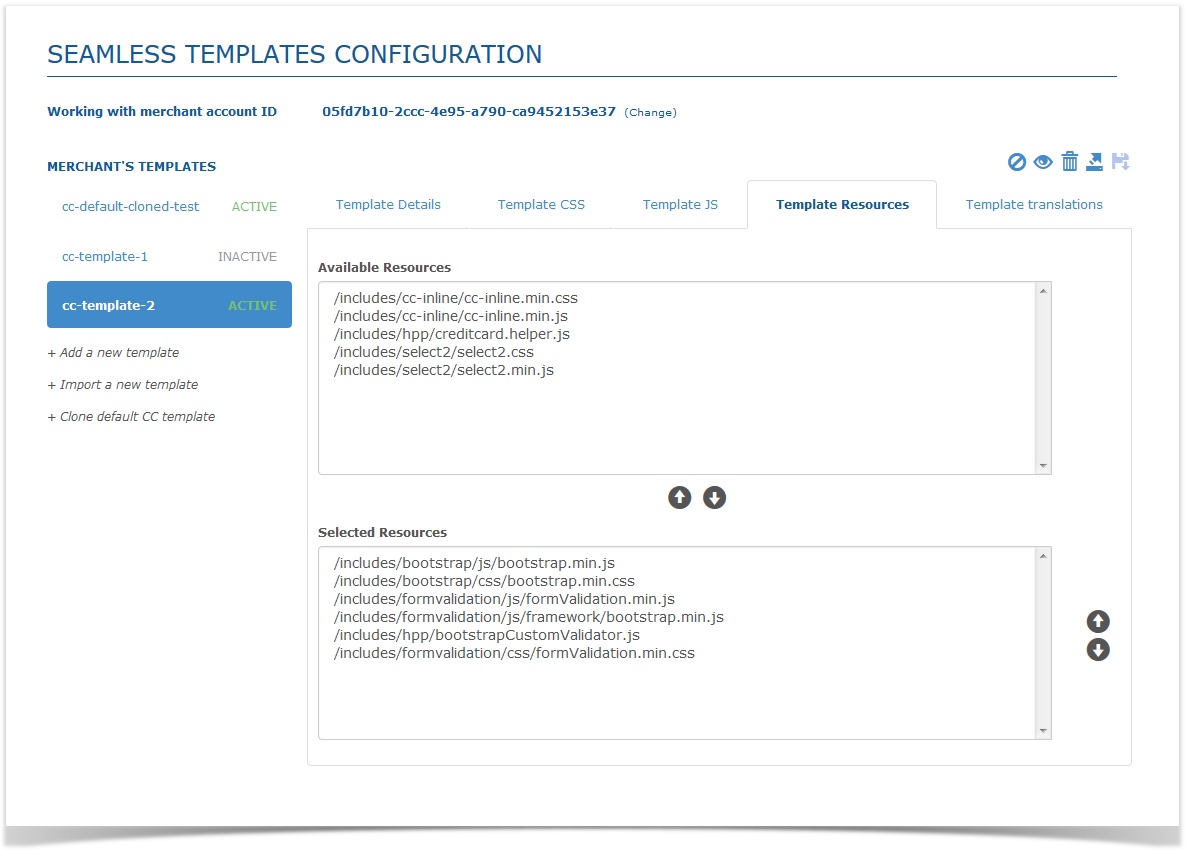
Translation Management
A template-specific i18n configuration can be made within the Template Translations tab. Here, new translations can be created or existing ones updated/deleted. Changes are applied once the whole template is successfully saved. The user can add/paste all required translations there (for multiple locales) at once.
Each i18n configuration must contain a language code (2-letters code in square brackets)
at the beginning followed by all required
key=value
translations mappings separated by a new line. The language
code is case-insensitive. There can be just one translation key per
locale. The translation key can contain letters, numerics, dashes
or underscores only.
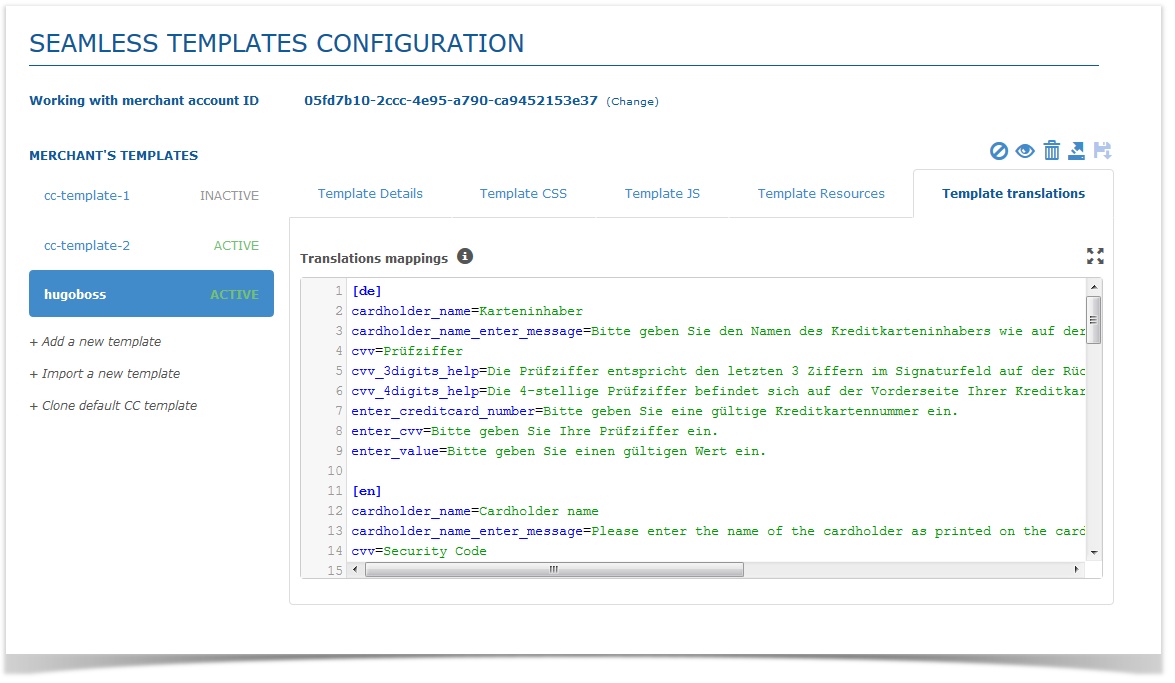
Seamless i18n
Translations are maintained by the jquery.i18n.properties
library and its
EE extension, ee.i18n
library. Each element containing a data-i18n
attribute is automatically translated according to the locale (2-letters
language code) specified within the payment request. The value of the
data-i18n
attribute represents a translation key. Please see the list of
current default translation keys and associated translations below.
They are used within the default Seamless template. It is possible to
override all these translations as well as specify the new ones when
configuring a new template. It is also possible to define an arbitrary
language code.
# default language (English)
card_number=Card number
expiry_date=Valid until
year=Year
month=Month
invalid_expiry_date=Please enter a valid expiry date
first_name=First name
last_name=Last name
enter_value=Please enter a value
select_valid_card_type=Please enter a valid credit card type
enter_creditcard_number=Please enter the valid and confirmed credit card number
enter_cvv=Please enter a valid security code (CVV)
[de]
card_number=Kartennummer
first_name=Vorname
last_name=Nachname
expiry_date=Gültig bis
year=Jahr
month=Monat
enter_value = Bitte geben Sie einen Wert ein
enter_creditcard_number = Bitte geben Sie die gültige und bestätigte Kreditkartennummer an
select_valid_card_type = Bitte geben Sie den gültigen Kreditkartentyp an
invalid_expiry_date = Bitte gültiges Ablaufdatum angeben
enter_cvv = Bitte geben Sie den gültigen Sicherheitscode (CVV) an
[es]
card_number=Número de tarjeta
first_name=Nombre
last_name=Apellidos
expiry_date=Válido hasta
year = Año
enter_value = Introduzca un valor
enter_creditcard_number = Indique una tarjeta de crédito válida y confirmada
select_valid_card_type = Indique un tipo de tarjeta de crédito válido
invalid_expiry_date = Indique una fecha de caducidad válida
enter_cvv = Indique el código de seguridad (CVV) válido
[fr]
card_number=Numéro de carte
first_name=Prénom
last_name=Nom
expiry_date=Date d''expiration
year = Année
enter_value = Veuillez saisir une valeur
enter_creditcard_number = Veuillez saisir un numéro de carte de crédit valide et confirmé
select_valid_card_type = Veuillez saisir un type de carte de crédit valide
invalid_expiry_date = Veuillez saisir une date d'expiration valide
enter_cvv = Veuillez saisir un code de sécurité valide (CVV)
[it]
card_number=Numero di carta
first_name=Nome
last_name=Cognome
expiry_date=Valido fino a
year = Anno
enter_value = Immettere un valore
enter_creditcard_number = Immettere un numero di carta di credito valido e confermato
select_valid_card_type = Immettere un tipo di carta di credito valido
invalid_expiry_date = Immettere una data di scadenza valida
enter_cvv = Immettere un codice di sicurezza valido (CVV)
[nl]
card_number=Kaartnummer
first_name=Voornaam
last_name=Achternaam
expiry_date=Geldig tot
year = Jaar
enter_value = Voer een waarde in
enter_creditcard_number = Voer een geldig en bevestigd creditcardnummer in
select_valid_card_type = Voer een geldig creditcardtype in
invalid_expiry_date = Voer een geldige einddatum in
enter_cvv = Voer de geldige veiligheidscode (CVV) in
Template PREVIEW
To preview the selected and saved template press the
button.
A couple of details need to be entered before the preview is shown - such as
dimensions, locale or currency (currency is necessary just in case the selected template needs the merchant’s configured card types model).
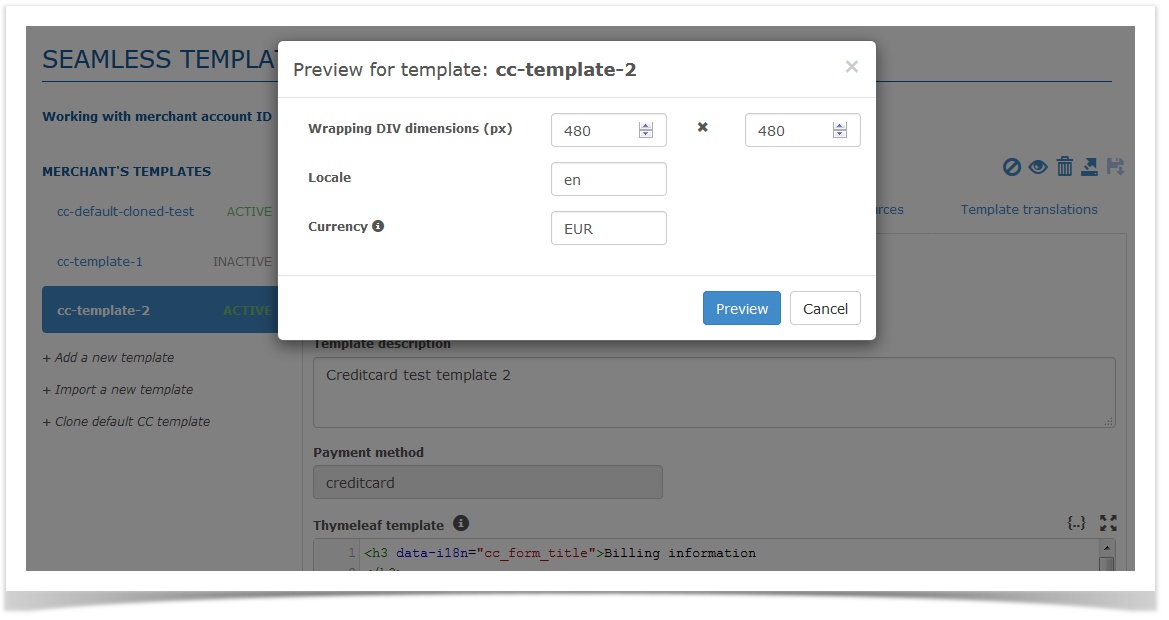
The template preview is provided by the endpoint
{URL}/engine/rest/seamless/renderform/preview/{merchantAccountId}/{paymentMethodId}/{templateName}
and handled by
com.ep.engine.controller.SeamlessPaymentController
When the appropriate GET
request is performed, the rendered template view
is returned and it looks exactly as if it would be rendered via
/engine/rest/seamless/renderform endpoint
This endpoint is secured by a basic authentication and the role
ROLE_CONFIG_SEAMLESS
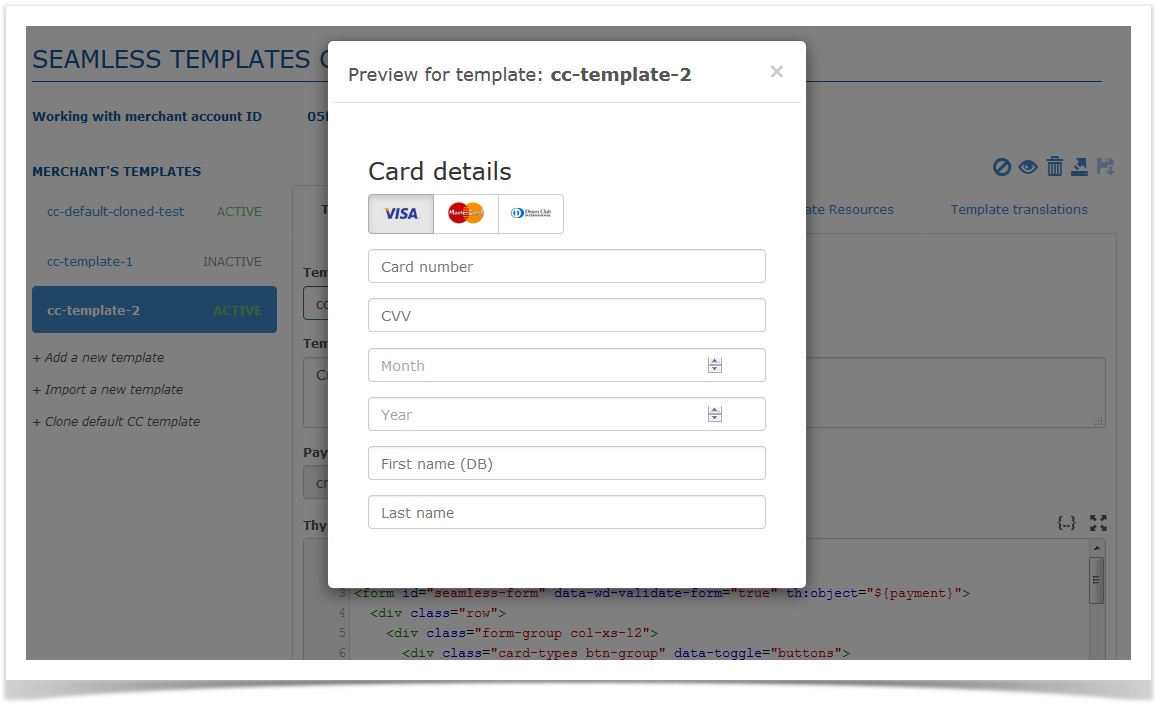
Import/Export Template
The selected template can be exported to a .json file by clicking the export button
.
The exported template can then be imported within different environments
(integration, test, production, …) by clicking on + Add a template > Import from JSON file
and selecting the particular .json file from the local storage.
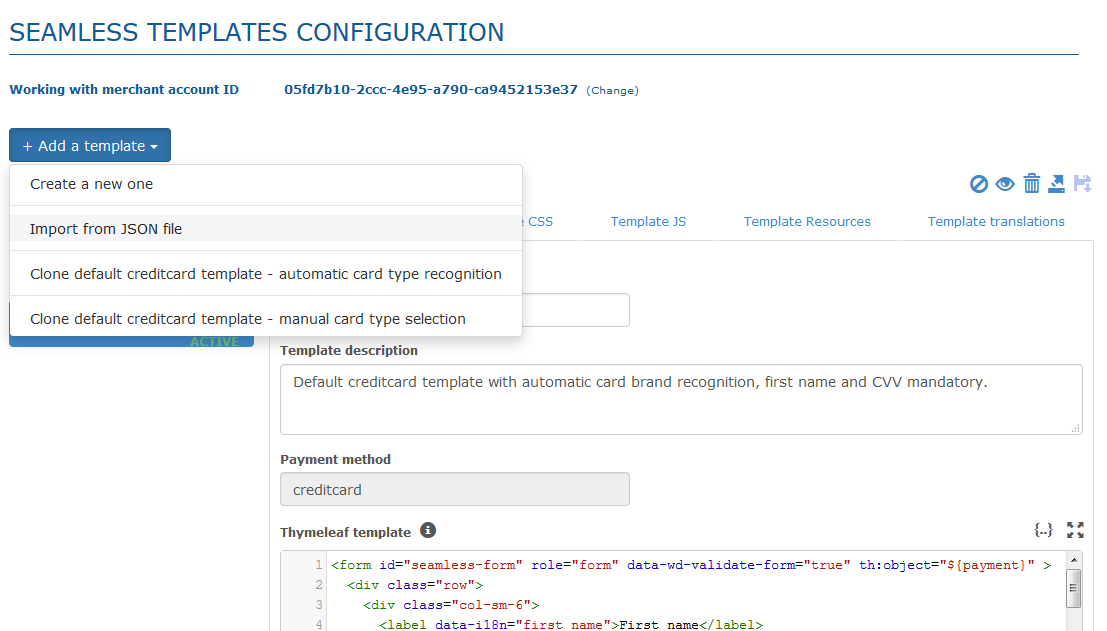
Clone Default Template
The default credit card template default-cc-template
can be cloned to
the current merchant account’s Seamless configuration by clicking on
+ Clone default CC template. This feature can help in situations when
just few changes within the default template would be needed. Once the
default template is cloned, the configuration can be finished much easier.
Default Seamless Template
Merchants have two options with which Seamless can be shown:
-
Manual Card Brand Selection
-
Automatic Card Brand Recognition (default)
If there is a need to use manual selection, please send template_name
in the request.
template_name = default-cc-template
template_name = default-cc-auto
Fields
Most of the fields from REST API are also available for Hosted Payment Page (HPP), Embedded Payment Page (EPP) and Seamless integration, differing only in the usage of an underscore instead of a hyphen.
The following table describes the fields that may appear in the HTML form in case of Hosted Payment Page (HPP) or as data for a JavaScript call in case of Embedded Payment Page (EPP) or Seamless integration.
Field Information | Description | ||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
|
Description |
||||||||||||||
|
The UTC time-stamp that represents the request. |
||||||||||||||
|
Refer to SHA-256 request signature. |
||||||||||||||
|
A unique identifier assigned for every Merchant Account. |
||||||||||||||
|
The unique string that the merchant sends with every transaction in order to uniquely identify it. The merchant system can subsequently request the status or existence of a transaction using this identifier. |
||||||||||||||
|
The method in which the account holder information was collected. Possible values are:
|
||||||||||||||
|
Indicates how and why a
payment occurs more than once. Possible values include |
||||||||||||||
|
Used in conjunction with
periodic_type to indicate the sequence. Possible values include |
||||||||||||||
|
A unique identifier assigned for every transaction type. |
||||||||||||||
|
The only amount that accompanies the transaction when it is created and/or requested. In the case of a sale or refund, this is what the merchant requests. In the case of a chargeback, this is the amount that is being contested. |
||||||||||||||
|
The currency in which a transaction is originally completed. |
||||||||||||||
|
The first name of the account holder. |
||||||||||||||
|
The last name of the account holder. |
||||||||||||||
|
A unique identifier assigned for every card token. This is a surrogate value for the primary account number. |
||||||||||||||
|
A card scheme accepted by the processing system. This includes physically issued cards. |
||||||||||||||
|
The embossed or encoded number that identifies the card issuer to which a transaction is to be routed and the account to which it is to be charged unless specific instructions indicate otherwise. In the case of a credit card, this is the primary account number. |
||||||||||||||
|
The 2-digit representation of the expiration month of the card account. |
||||||||||||||
|
The 4-digit representation of the expiration year of the card account. |
||||||||||||||
|
A security feature for credit or debit card transactions, providing increased protection against credit card fraud. The Card Security Code is located on the back of Mastercard, Visa and Discover credit or debit cards and is typically a separate group of 3 digits to the right of the signature strip. On American Express cards, the Card Security Code is a printed, not embossed, group of four digits on the front towards the right. |
||||||||||||||
|
The URL where the account holder will be redirected to following transaction completion. |
||||||||||||||
|
The IP address of the cardholder as recorded by the entity receiving the transaction attempt from the cardholder. |
||||||||||||||
|
The email address of the account holder. |
||||||||||||||
|
The phone number of the account holder. |
||||||||||||||
|
Merchant-provided string to store the order detail for the transaction. |
||||||||||||||
|
Merchant-provided string to store the order detail for the transaction. |
||||||||||||||
|
The merchant CRM ID for the account holder. |
||||||||||||||
|
Text used to name the transaction custom field. Possible values for n can be in the range from 1 to 10. |
||||||||||||||
|
Used with a key, the content used to define the value of the transaction custom field. Possible values for n can be in the range from 1 to 10. |
||||||||||||||
|
WPG uses the notification URL to inform the merchant about the outcome of the payment process. Usually the merchant provides one URL, to which WPG will send the notification to. If required, the merchant can define more than one notification URL. |
||||||||||||||
|
The merchants can provide notification URLs which correspond to the
transaction state. The merchants define one URL for success and one for failure. |
||||||||||||||
|
The field which is shown on the customer’s card statement. This feature is not supported by all the acquirers. The size of this field depends on the acquirer. Please contact technical support for further clarification. |
||||||||||||||
|
A unique identifier assigned for every parent transaction. |
||||||||||||||
|
Text used to name the payment method. |
||||||||||||||
|
Code to indicate which default language the payment page should be rendered in. |
||||||||||||||
|
A device fingerprint is information collected about a remote computing device for the purpose of identification. Fingerprints can be used to fully or partially identify individual users or devices even when cookies are turned off. |
||||||||||||||
|
The URL to which the Account Holder will be re-directed during payment processing. This is normally a page on the Merchant’s website. |
||||||||||||||
|
The URL to which the Account Holder will be re-directed after he has cancelled a payment. This is normally a page on the Merchant’s website. |
||||||||||||||
|
The URL to which the Account Holder will be re-directed after an unsuccessful payment. This is normally a page on the Merchant’s website notifying the Account Holder of a failed payment often with the option to try another Payment Method. |
||||||||||||||
|
The URL to which the Account Holder will be re-directed after a successful payment. This is normally a success confirmation page on the Merchant’s website. |
||||||||||||||
|
Configuration specific category name for automatic merchant account resolving based on logged in user or "super merchant account". |
||||||||||||||
|
This field
has been replaced by |
||||||||||||||
|
Cryptogram type enumeration – |
||||||||||||||
|
Cryptogram value for android or apple creditcard payments. |
||||||||||||||
|
The signature of the Mandate Transaction. |
||||||||||||||
|
The city that the Mandate was signed in. |
||||||||||||||
|
The date that the Mandate was signed. |
||||||||||||||
|
The date that the Mandate Transaction is due. |
||||||||||||||
|
The Mandate ID for the Mandate Transaction. |
||||||||||||||
|
|||||||||||||||
|
The national bank sorting code for national bank transfers. |
||||||||||||||
|
The name of the consumer’s bank. |
||||||||||||||
|
The number designating a bank account used nationally. |
||||||||||||||
|
Bank account owner name (not used anymore - |
||||||||||||||
|
The International Bank Account Number required in a Bank Transfer. It is an international standard for identifying bank accounts across national borders. The current standard is ISO 13616:2007, which indicates SWIFT as the formal registrar. |
||||||||||||||
|
The Bank Identifier Code information required in a Bank Transfer. |
||||||||||||||
|
The city that the bank is located in. Typically required for Chinese Bank Transfers. |
||||||||||||||
|
The state that the bank is located in. Typically required for Chinese Bank Transfers. |
||||||||||||||
|
The address of the bank. Typically required for Chinese Bank Transfers. |
||||||||||||||
|
ZIP postal Code |
||||||||||||||
|
Account holder country code. |
||||||||||||||
|
Payment related country code – usually used for payment method specific validation (country restrictions) |
||||||||||||||
|
State |
||||||||||||||
|
City |
||||||||||||||
|
Primary Street Address |
||||||||||||||
|
Secondary Street Address |
||||||||||||||
|
The Social Security number of the Account Holder. |
||||||||||||||
|
URL of payment method provider that user should be redirected to so payment can be finished. (not needed and ignored in request.) |
||||||||||||||
|
Indicates that the Transaction Request should proceed with the 3D Secure workflow if the Card Holder is enrolled. Otherwise, the transaction proceeds without 3D Secure. This field is used in conjunction with Hosted Payment Page. |
||||||||||||||
|
The assigned skin name for a merchant’s customized HPP skin. This will display the merchant’s skin instead of the default skin. |
||||||||||||||
|
Not needed in request. EE internal Payment Service Provider ID. |
||||||||||||||
|
Batch payment… |
||||||||||||||
|
Batch payment… |
||||||||||||||
|
The Identifier of the Consumer. |
||||||||||||||
|
Consumer email address. |
||||||||||||||
|
|||||||||||||||
|
Reserved for future use. |
||||||||||||||
|
Common ID for all referenced transactions. It is usually transaction ID of the first transaction in chain. |
||||||||||||||
|
Content type of the IPN (application/xml, application/json, application/x-www-form-urlencoded). |
||||||||||||||
|
Account holder birth date. |
||||||||||||||
|
The Creditor Id for the Merchant Account SEPA. |
||||||||||||||
|
Enumeration – |
||||||||||||||
|
The Country ID portion of the address of the Shipping Address. |
||||||||||||||
|
The state or province portion of the address of the Shipping Address. |
||||||||||||||
|
The city of the address of the Shipping Address. |
||||||||||||||
|
The postal code or ZIP of the address of the Shipping Address. |
||||||||||||||
|
The first line of the street address of the Shipping Address. |
||||||||||||||
|
The second line of the street address of the Shipping Address. |
||||||||||||||
|
The first name of the Shipping Address. |
||||||||||||||
|
The last name of the Shipping Address. |
||||||||||||||
|
Additional shipping information (paylah). |
||||||||||||||
|
Additional shipping information (paylah). |
||||||||||||||
|
Additional shipping information (paylah). |
||||||||||||||
|
The phone number of the Shipping Address. |
||||||||||||||
|
Digitally signed, base64-encoded authentication response message received from the issuer (3D Secure transaction). |
||||||||||||||
|
URL specified by merchant pointing to the CSS resource customizing HPP/EPP. |
||||||||||||||
|
Order item name. |
||||||||||||||
|
Order item article identifier. |
||||||||||||||
|
Order item amount. |
||||||||||||||
|
Order item quantity. |
||||||||||||||
|
Order item description. |
||||||||||||||
|
One time password (icashcard). |
||||||||||||||
|
The unique identifier of the Account Holder’s Wallet Account. |
||||||||||||||
|
IP address of consumer obtained by payment page in time of payment. |
||||||||||||||
|
Consumer’s web browser obtained by payment page in time of payment. |
||||||||||||||
|
Version number of consumer’s web browser obtained by payment page in time of payment. |
||||||||||||||
|
Consumer’s operating system obtained by payment page in time of payment. |
||||||||||||||
|
Consumer’s screen resolution obtained by payment page in time of payment. |
||||||||||||||
|
URL referring to previous page consumer visited before payment page. |
||||||||||||||
|
Indicating liability shift in case of 3D Secure transactions. Possible values: Y - Liability Shift transferred to issuer |
||||||||||||||
|
Consumer date of birth. |
||||||||||||||
|
Social security number of the consumer. |
||||||||||||||
|
Gender of consumer. |
||||||||||||||
|
Order item(s) price(s) per unit. |
||||||||||||||
|
Total count(s) of the item(s) in the order. |
||||||||||||||
|
Item EAN(s) or other article(s) identifier(s). |
||||||||||||||
|
Name(s) of the item(s) in the basket. |
||||||||||||||
|
Currency(ies) of the order item amount(s). |
||||||||||||||
|
Order item tax rate(s) in percentage already included within order item price ( |
||||||||||||||
|
Order item tax(es) per unit already included within the order item price ( |
||||||||||||||
|
Order item name. |
||||||||||||||
|
Order item article identifier. |
||||||||||||||
|
Order item price. |
||||||||||||||
|
Currency of the order item price ( |
||||||||||||||
|
Order item tax rate in percentage already included within order item price ( |
||||||||||||||
|
Order item tax per unit already included within the order item price ( |
||||||||||||||
|
Tax amount currency. |
||||||||||||||
|
Total count of the item in the order. |
||||||||||||||
|
The airline code assigned by IATA. |
||||||||||||||
|
Name of the airline. |
||||||||||||||
|
The file key of the Passenger Name Record (PNR). This information is mandatory for transactions with AirPlus UATP cards. |
||||||||||||||
|
The name of the Airline Transaction passenger. |
||||||||||||||
|
The phone number of the Airline Transaction passenger. |
||||||||||||||
|
The Email Address of the Airline Transaction passenger. |
||||||||||||||
|
The IP Address of the Airline Transaction passenger. |
||||||||||||||
|
The date the ticket was issued. |
||||||||||||||
|
The airline ticket number, including the check digit. If no airline ticket number (IATA) is used, the element field must be populated with 99999999999. |
||||||||||||||
|
Indicates that the Airline Transaction is restricted. 0 = No restriction, 1 = Restricted (non-refundable). |
||||||||||||||
|
The Passenger Name File ID for the Airline Transaction. |
||||||||||||||
|
The airline ticket check digit. |
||||||||||||||
|
The agency code assigned by IATA. If no IATA code is used, the element field must be populated with 99999999. |
||||||||||||||
|
The agency name. |
||||||||||||||
|
This field must contain the net amount of the purchase transaction in the specified currency for which the tax is levied. Two decimal places are implied. If this field contains a value greater than zero, the indicated value must differ to the content of the transaction amount. |
||||||||||||||
|
The Issuer Address Street for the Airline Transaction. |
||||||||||||||
|
The Issuer Address Street 2 for the Airline Transaction. |
||||||||||||||
|
The city of the address of the Airline Transaction issuer. |
||||||||||||||
|
The state of the address of the Airline Transaction issuer. |
||||||||||||||
|
The Issuer Address Country ID for the Airline Transaction. |
||||||||||||||
|
An alphanumeric numeric code used to represent the Airline Transaction issuer Postal. |
||||||||||||||
|
The number of passengers on the Airline Transaction. |
||||||||||||||
|
The reservation code of the Airline Transaction passenger. |
||||||||||||||
|
The 2-letter airline code (e.g. LH, BA, KL) supplied by IATA for each leg of a flight. |
||||||||||||||
|
The departure airport code. IATA assigns the airport codes. |
||||||||||||||
|
The departure City Code of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The arrival airport code of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The arrival city code of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The departure date for a given leg. |
||||||||||||||
|
The arrival date of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The flight number of the Itinerary Segment. |
||||||||||||||
|
Used to distinguish between First Class, Business Class and Economy Class, but also used to distinguish between different fares and booking codes within the same type of service. |
||||||||||||||
|
Represents a specific fare and class of service with letters, numbers, or a combination of both. |
||||||||||||||
|
0 = allowed, 1 = not allowed |
||||||||||||||
|
The amount of the Value Added Tax levied on the transaction amount in the specified currency. |
||||||||||||||
|
The airline code assigned by IATA. |
||||||||||||||
|
The agency code assigned by IATA. |
||||||||||||||
|
This indicates if the package includes car rental, airline flight, both
or neither. Valid entries include: |
||||||||||||||
|
The ticket number, including the check digit. |
||||||||||||||
|
The name of the passenger. |
||||||||||||||
|
The cruise departure date also known as the sail date. |
||||||||||||||
|
The cruise return date also known as the sail end date. |
||||||||||||||
|
The total cost of the cruise. |
||||||||||||||
|
The length of the cruise in days. |
||||||||||||||
|
The ship name booked for the cruise. |
||||||||||||||
|
The name of the city where the lodging property is located. |
||||||||||||||
|
The region code where the lodging property is located. |
||||||||||||||
|
The country code where the lodging property is located. |
||||||||||||||
|
The 2-letter airline code (e.g. LH, BA, KL) supplied by IATA for each leg of a flight. |
||||||||||||||
|
The departure airport code. IATA assigns the airport codes. |
||||||||||||||
|
The departure City Code of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The arrival airport code of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The arrival city code of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The departure date for a given leg. |
||||||||||||||
|
The arrival date of the Itinerary Segment. IATA assigns the airport codes. |
||||||||||||||
|
The flight number of the Itinerary Segment. |
||||||||||||||
|
Used to distinguish between First Class, Business Class and Economy Class, but also used to distinguish between different fares and booking codes within the same type of service. |
||||||||||||||
|
Represents a specific fare and class of service with letters, numbers, or a combination of both. |
||||||||||||||
|
0 = allowed, 1 = not allowed |
||||||||||||||
|
The amount of the Value Added Tax levied on the transaction amount in the specified currency. |
||||||||||||||
|
This field uses cardinal numbers which are treated like a string. It determines the timeout of an HPP page in milliseconds when this HPP page uses popup. During an open popup, Wirecard Payment Gateway polls query requests. If these poll requests are not accomplished until timeout, polling stops and displays a message. Default timeout is 10 minutes (value = "600000"). |
Field Information | Description |
---|---|
|
Description |
|
Refer to SHA-256 response signature. |
|
A unique identifier assigned for every transaction type. |
|
The current status of a transaction. Typically, a transaction will start from a submitted state, to an in-progress, and then finish in either the success or failed state. |
|
A unique identifier assigned for every transaction. |
|
The unique string that the merchant sends with every transaction in order to uniquely identify it. The merchant system can subsequently request the status or existence of a transaction using this identifier. |
|
The only amount that accompanies the transaction when it is created and/or requested. In the case of a sale or refund, this is what the merchant requests. In the case of a chargeback, this is the amount that is being contested. |
|
A unique identifier assigned for every Merchant Account. |
|
The UTC time-stamp that represents the response. |
|
The status of a transaction. This is primarily used in conjunction with the transaction state to determine the exact details of the status of the transaction. |
|
Text used to describe the transaction status. |
|
The severity of the transaction, can be information, warning, error. |
|
The unique identifier for a provider transaction, typically generated by the provider. |
|
Provider’s reference ID. This may be non-unique. |
|
An alphanumeric numeric code used to represent the provider authorization. |
|
A unique identifier assigned for every card token. This is a surrogate value for the primary account number. |
|
A code used to represent the card masked account. |
|
The IP address of the cardholder as recorded by the entity receiving the transaction attempt from the cardholder. |